Hello people, this is a simple Java program to find out the number of words in a sentence. Very useful for high school / college students
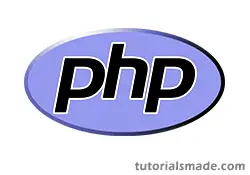
Palindrome number is a number if you reverse the number you will get the same result, for eg., below are the palindrome numbers: 898, 909, 12321 So,
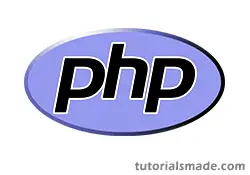
This sample program is very helpful for the School / College kids who are willing to become a PHP developer. And in the interviews these kind
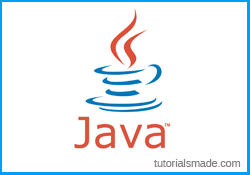
A simple Java program to find Largest and Smallest number in a Java List. Let’s see the program first,
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.ArrayList; import java.util.List; /** * * @author agurchand */ public class LargeSmallNumber { /** * @param args the command line arguments */ public static void main(String[] args) throws IOException { //create buffer to capture input BufferedReader in = new BufferedReader(new InputStreamReader(System.in)); //define list and integers List list = new ArrayList(); int len, largest, smallest, number; //get the limit from user System.out.println("Enter the limit (Better give below 10): "); len = Integer.parseInt(in.readLine()); //get the list from user System.out.println("Now enter the list:"); for (int i = 0; i < len; i++) { list.add(Integer.parseInt(in.readLine())); } //assign the first value of list into largest, smallest largest = (int) list.get(0); smallest = (int) list.get(0); //now loop through each list and find largest and smallest number in the list for (Object list1 : list) { number = (int) list1; if (number > largest) { largest = number; } else if (number < smallest) { smallest = number; } } System.out.println("Largest Number is : " + largest); System.out.println("Smallest Number is : " + smallest); } } |
Read the comments in the above
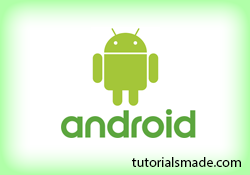
There are some requirements you may need : 1. Your phone should have Android 4.4 Kitkat. 2. ADB drivers should be installed on your system.
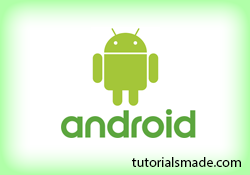
To enable USB Debugging mode on your android phone, follow the instructions: 1. Go to settings and click About: 2. Scroll to the Build number and
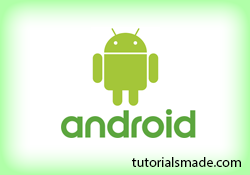
This is so far the smallest ADB Drivers I found in the Internet developed by Snoop05 Features: 1. Installs in less than 15 seconds. 2. Size
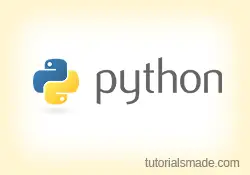
To print timestamp in Python is very simple, all you have to do is, import the time library and call the time() function to get
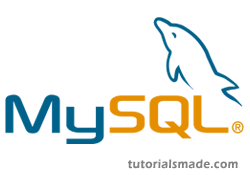
The answer for this question is very simple if you already have experience in multiple SQL Servers such as MySQL, MS SQL 2005, PostgreSQL etc., But,
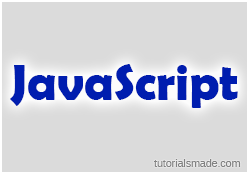
In this post you are going to learn how to create an array variable and push values into that array in JavaScript. How to create