Let’s write a simple Java program to generate a multiplication table. It’s fairly a simple program. I am going to write this as a dynamic
Category: Java
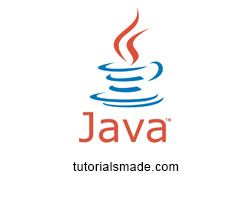
Let’s see an interesting program for school/college students who are learning core Java. To find whether an entered number is Odd or Even is very
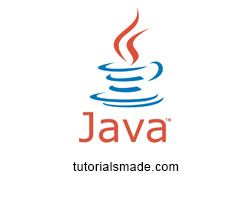
Hi friends, In this post, we are going to discuss how to print a series of Prime numbers using Core Java. This basic Java program
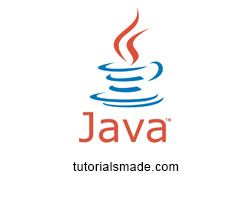
In this post, we are going to write a simple Java Program to find the Quotient & Remainder. Before writing the program, let’s see what
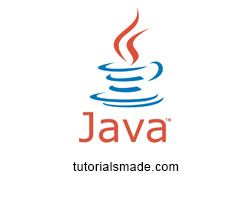
Here is a simple Java program for beginners, Write a Java program to find a leap year or not. Formula for finding a Leap year
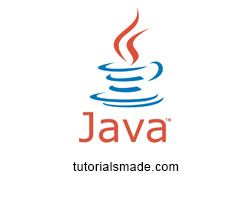
Let’s see how to write a simple Java program to calculate the factorial If you don’t know what is factorial, let me show you!! Factorial
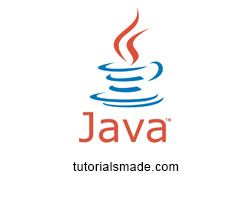
Printing alphabets in Java Programming? Oh common. It may look silly but you know many of us still don’t know how to write a program
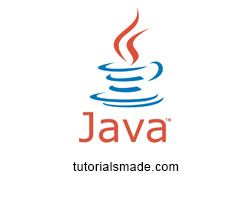
Hello friends and dear programmers, today we are going to write a Java program which will count the number of occurrence of a character
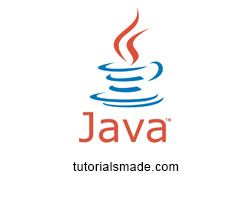
Hello people, this is a simple Java program to find out the number of words in a sentence. Very useful for high school / college students
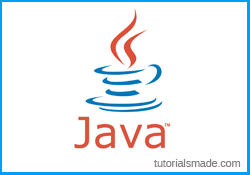
A simple Java program to find Largest and Smallest number in a Java List. Let’s see the program first,
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.ArrayList; import java.util.List; /** * * @author agurchand */ public class LargeSmallNumber { /** * @param args the command line arguments */ public static void main(String[] args) throws IOException { //create buffer to capture input BufferedReader in = new BufferedReader(new InputStreamReader(System.in)); //define list and integers List list = new ArrayList(); int len, largest, smallest, number; //get the limit from user System.out.println("Enter the limit (Better give below 10): "); len = Integer.parseInt(in.readLine()); //get the list from user System.out.println("Now enter the list:"); for (int i = 0; i < len; i++) { list.add(Integer.parseInt(in.readLine())); } //assign the first value of list into largest, smallest largest = (int) list.get(0); smallest = (int) list.get(0); //now loop through each list and find largest and smallest number in the list for (Object list1 : list) { number = (int) list1; if (number > largest) { largest = number; } else if (number < smallest) { smallest = number; } } System.out.println("Largest Number is : " + largest); System.out.println("Smallest Number is : " + smallest); } } |
Read the comments in the above