Let’s write a JavaScript program to print Even Numbers within a given range. First, to find an Even number it is very simple, divide the
Category: JavaScript
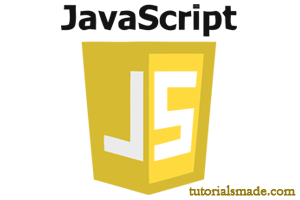
Let’s write a JavaScript program to print Odd Numbers within a given range. First, to find an Odd number it is very simple, divide the
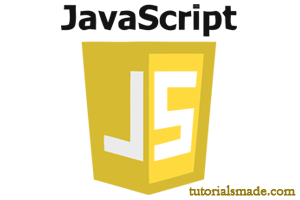
Here in this post, we are going to see how to convert Binary numbers to Decimal numbers. To convert any Binary number to Decimal in
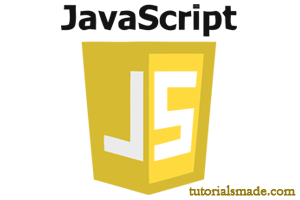
To find the Power of a number in JavaScript you can use the readily available function Math.pow(). But sometimes, especially in the interviews they might
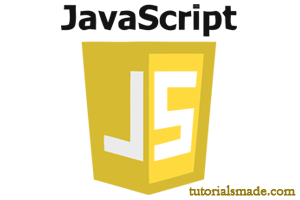
In this post, we are going to write a pure JavaScript program to print alphabets from A to Z and a to z. To print
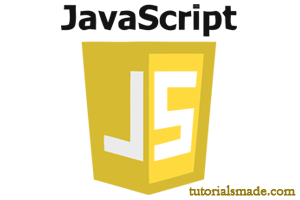
In this post we are going to write a JavaScript program to find the “Area of Triangle”. Here is the complete JavaScript program to find
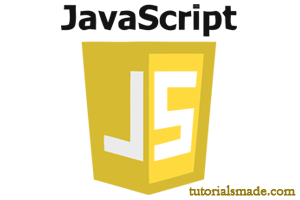
Hello students and self-learners, here is another interesting JavaScript program for you to learn the fundamentals. In this post, I am going to write a
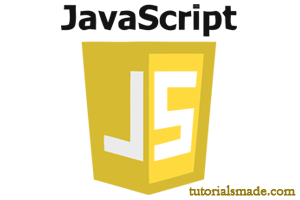
In this post, you will be learning how to do Addition, Subtraction, Multiplication & Division using pure JavaScript. Let’s see a simple snippet first:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
//addition add = 5 + 2; document.write(add+"<br>"); //subtraction sub = 5 - 2; document.write(sub+"<br>"); //multiplication mul = 5 * 2; document.write(mul+"<br>"); //division div = 5 / 2; document.write(div+"<br>"); |
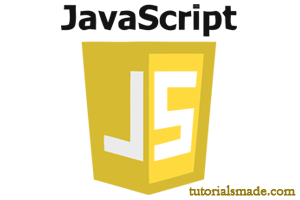
This post will explain how to find a substring in a string using pure JavaScript. Let’s see how can we achieve this.
1 2 3 |
var str = 'testing'; var substr = 'tin'; alert(str.indexOf(substr) !== -1); |
The above
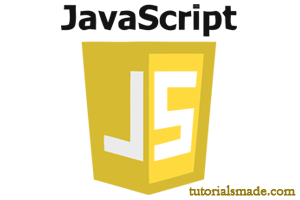
JavaScript is one of mostly used scripting language all over the world. Today in this post we are going to see how to write a