In this post, we are going to see how to write a simple JavaScript program to generate a Multiplication table. The output will generate something
Category: JavaScript
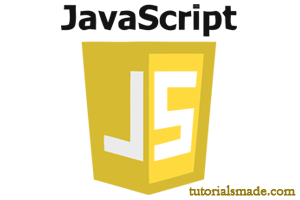
To play sounds in JavaScript, you can use the HTML5 Audio API. Here is a basic example of how to use it to play a
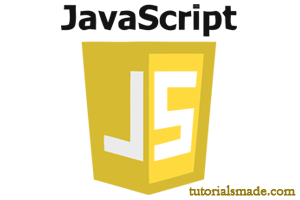
Here’s a simple program in JavaScript that can help you find the IP address of the device running the code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
<html> <head> <title>Find your IP Address using JavaScript</title> <script> // using api.ipify.org to get the public ip address // using JavaScript Fetch API to perform HTTP Request fetch('https://api.ipify.org?format=json') .then(response => response.json()) .then(data => document.getElementById("response").innerHTML = data.ip) .catch(error => console.error(error)); </script> </head> <body> Your Public IP Address is: <span id="response"></span> </body> </html> |
This program uses the
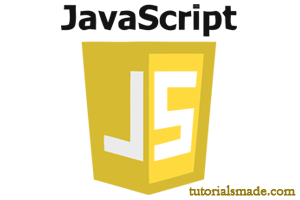
In this post, I am will show you to write a simple JavaScript function to print Floyd’s Triangle pattern. If you don’t know what is
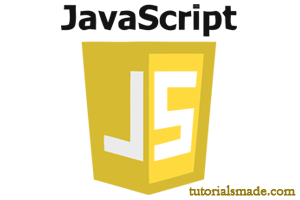
In this post, we are going to see how to write a JavaScript countdown timer. Here is the JavaScript countdown timer script for you,
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
//JS code for countdown timer function countdownTimer() { const difference = +new Date('2023-10-11') - +new Date(); let remaining = "Time's up!"; if (difference > 0) { const parts = { days: Math.floor(difference / (1000 * 60 * 60 * 24)), hours: Math.floor((difference / (1000 * 60 * 60)) % 24), minutes: Math.floor((difference / 1000 / 60) % 60), seconds: Math.floor((difference / 1000) % 60) }; remaining = Object.keys(parts) .map(part => { if (!parts[part]) return; return `${parts[part]} ${part}`; }) .join(" "); } document.getElementById("countdown").innerHTML = remaining; } |
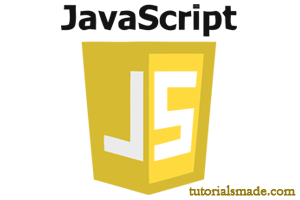
In this post, I will show you how to write a simple JavaScript program to check if a number is Negative or Positive. I am
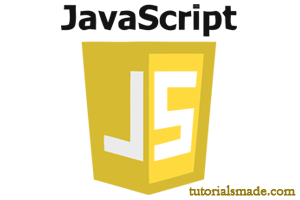
In this post, I am going to show you how to write a simple JavaScript program to convert Kilometers to Miles. The formula is very
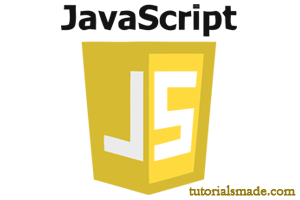
In this post, we are going to see how to print Prime numbers between two intervals in JavaScript. This program also helps you to understand
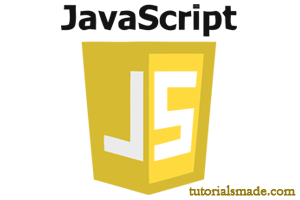
Before discussing the main topic, let us see how to print a random number in plain JavaScript. If you don’t know already there is this
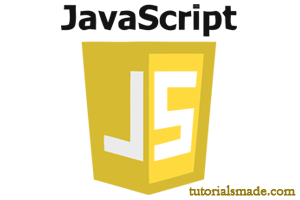
Let’s write a simple JavaScript to count the number of vowels present in a string and print it. And also you will learn the below